PostgreSQL UNNEST: Complete Array Function Guide [2024]
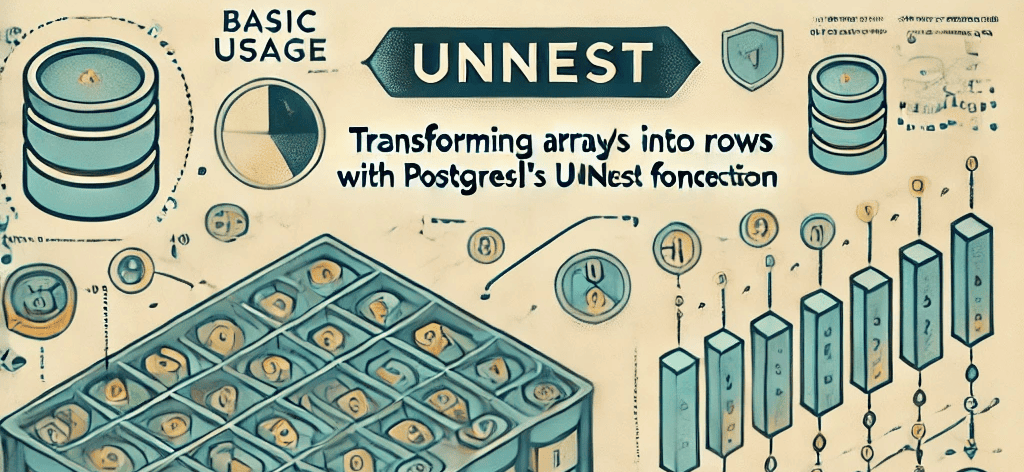
PostgreSQL's UNNEST function transforms array elements into rows, providing a powerful way to work with array data in a relational format. This comprehensive guide covers everything from basic usage to advanced techniques and performance optimization.
Table of Contents
- Introduction
- Basic Concepts
- UNNEST vs IN Comparison
- Real-World Examples
- Performance Optimization
- Advanced Usage
- Best Practices
- Troubleshooting
Introduction
UNNEST is a specialized PostgreSQL function that converts array elements into individual rows. This transformation is essential for:
- Converting array data into queryable rows
- Performing complex array operations
- Analyzing array elements individually
- Joining array data with other tables
Basic Concepts
Simple Array Unnesting
-- Basic array unnesting SELECT unnest(ARRAY['apple', 'banana', 'orange']); -- Result: -- unnest -- -------- -- apple -- banana -- orange
UNNEST with Column Alias
SELECT unnest(ARRAY[1, 2, 3]) AS numbers; -- Result: -- numbers -- --------- -- 1 -- 2 -- 3
UNNEST vs IN Comparison
Understanding when to use UNNEST versus IN is crucial for efficient queries.
Using IN
-- Traditional IN clause SELECT * FROM products WHERE category IN ('Electronics', 'Gaming');
Using UNNEST
-- UNNEST approach SELECT * FROM products WHERE category = ANY(UNNEST(ARRAY['Electronics', 'Gaming']));
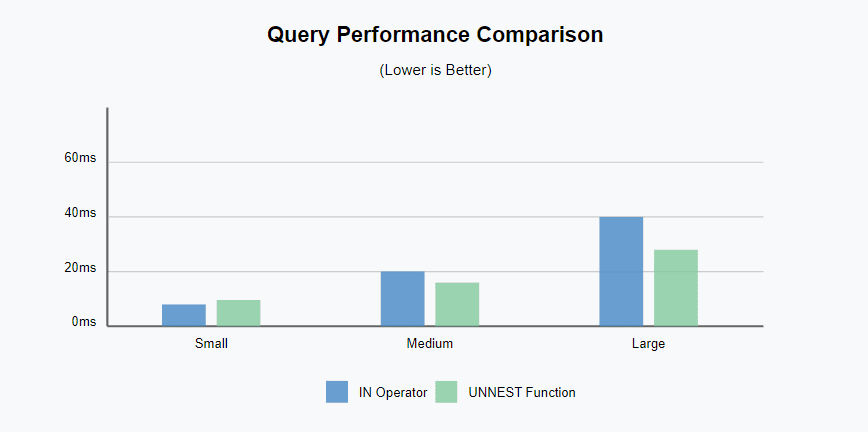
Key Differences
- Array Handling
- IN: Works with comma-separated values
- UNNEST: Native array support
- Performance
- IN: Better for small, fixed lists
- UNNEST: Efficient for array columns and dynamic data
- Flexibility
- IN: Limited to simple comparisons
- UNNEST: Supports complex array operations
Real-World Examples
E-commerce Product Tags System
-- Create products table CREATE TABLE products ( product_id SERIAL PRIMARY KEY, name VARCHAR(100), price DECIMAL(10,2), tags TEXT[] ); -- Insert sample data INSERT INTO products (name, price, tags) VALUES ('Gaming Laptop', 1299.99, ARRAY['electronics', 'gaming', 'computers']), ('Wireless Mouse', 29.99, ARRAY['electronics', 'accessories', 'gaming']); -- Find products by tag SELECT DISTINCT p.name, p.price FROM products p CROSS JOIN unnest(p.tags) AS t(tag) WHERE t.tag = 'gaming';
User Interests Analytics
-- Create users table CREATE TABLE users ( user_id SERIAL PRIMARY KEY, username VARCHAR(50), interests TEXT[] ); -- Insert sample data INSERT INTO users (username, interests) VALUES ('john_doe', ARRAY['technology', 'photography', 'travel']), ('jane_smith', ARRAY['travel', 'cooking', 'technology']); -- Find common interests WITH user_interests AS ( SELECT username, unnest(interests) as interest FROM users ) SELECT a.username as user1, b.username as user2, a.interest as shared_interest FROM user_interests a JOIN user_interests b ON a.interest = b.interest AND a.username < b.username;
Performance Optimization
1. Indexing Strategies
-- GIN index for array columns CREATE INDEX idx_product_tags ON products USING GIN (tags); -- Materialized view for frequently accessed unnested data CREATE MATERIALIZED VIEW product_tags AS SELECT product_id, unnest(tags) as tag FROM products; CREATE INDEX idx_product_tags_tag ON product_tags(tag);
2. Batch Processing
-- Process large arrays in batches WITH RECURSIVE batch_processor AS ( SELECT array_position(tags, unnest(tags)) as pos, unnest(tags) as tag FROM products WHERE product_id = 1 ) SELECT * FROM batch_processor ORDER BY pos;
Advanced Usage
UNNEST with Multiple Arrays
SELECT u1.elem AS array1_elem, u2.elem AS array2_elem FROM unnest(ARRAY[1, 2, 3]) WITH ORDINALITY AS u1(elem, ord) FULL OUTER JOIN unnest(ARRAY['a', 'b']) WITH ORDINALITY AS u2(elem, ord) ON u1.ord = u2.ord;
UNNEST with JSON Arrays
-- Convert JSON array to rows SELECT unnest(array( SELECT json_array_elements_text('["data1", "data2", "data3"]') )) AS json_items;
Best Practices
1. Order Preservation
-- Use WITH ORDINALITY to maintain array order SELECT * FROM unnest(ARRAY['apple', 'banana', 'orange']) WITH ORDINALITY AS t(fruit, position);
2. NULL Handling
-- Explicit NULL handling SELECT unnest(ARRAY[1, NULL, 3]) as numbers WHERE numbers IS NOT NULL;
3. Type Consistency
-- Ensure consistent data types SELECT unnest(ARRAY['1', '2', '3']::int[]) as numbers;
Troubleshooting
Common Issues and Solutions
1. Type Mismatch Errors
-- Solution: Explicit type casting SELECT unnest( ARRAY[CAST('2024-01-01' AS DATE), CAST('2024-01-02' AS DATE)] ) as dates;
2. Performance with Large Arrays
-- Solution: Batch processing SELECT unnest(your_array_column) FROM your_table WHERE array_length(your_array_column, 1) < 1000;
Error Handling Function
-- Safe unnest function with NULL handling CREATE OR REPLACE FUNCTION safe_unnest(arr anyarray) RETURNS TABLE (element anyelement) AS $$ BEGIN IF arr IS NULL THEN RETURN; END IF; RETURN QUERY SELECT unnest(arr); END; $$ LANGUAGE plpgsql;
Conclusion
PostgreSQL's UNNEST function is a versatile tool that simplifies array operations and data transformation. Key takeaways include:
- Use UNNEST for efficient array-to-row conversion
- Consider performance optimizations for large arrays
- Leverage WITH ORDINALITY when order matters
- Choose between IN and UNNEST based on use case
- Implement proper error handling and type checking
Last Updated: November 2024