Drizzle ORM: A Type-Safe and Modern ORM for TypeScript Applications
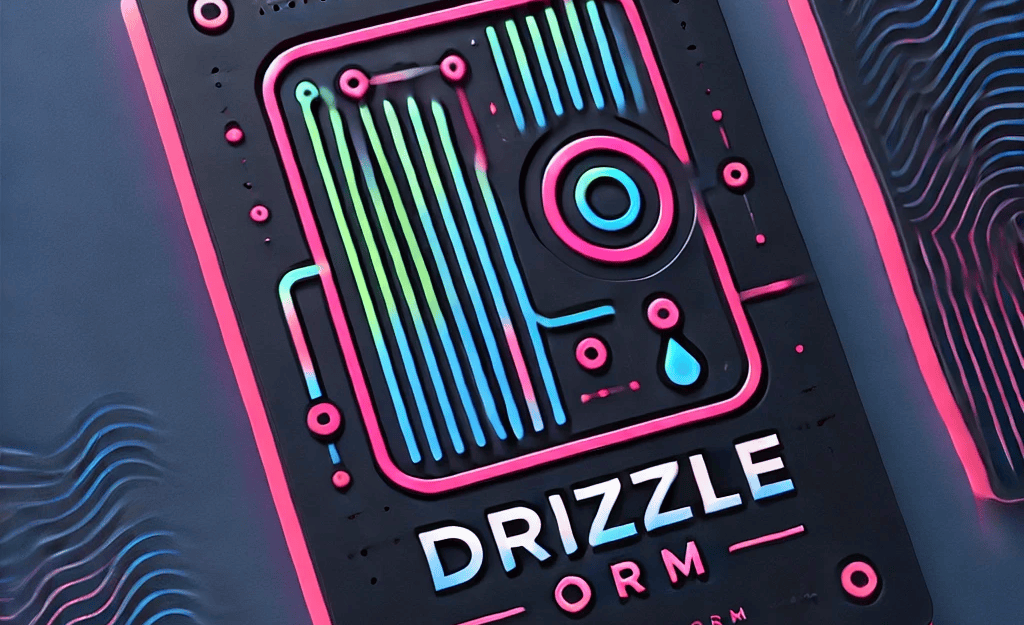
Introduction: What is Drizzle ORM?
Drizzle ORM is a lightweight, type-safe Object-Relational Mapping (ORM) solution specifically designed for TypeScript applications. It stands out in the ecosystem by providing a perfect balance between developer experience and performance, making it an ideal choice for modern web applications.
Key Features and Benefits
Type Safety at its Core
- Complete TypeScript integration
- Compile-time error detection
- Auto-completion support in modern IDEs
- Zero runtime type checking overhead
Performance-First Approach
- Minimal query overhead
- Direct SQL query translation
- Efficient connection pooling
- Optimized query planning
Developer Experience
- SQL-like query builder syntax
- Intuitive schema declarations
- Minimal learning curve for SQL developers
- Rich ecosystem of tools and plugins
Getting Started with Drizzle ORM
Installation Process
# Install core dependencies npm install drizzle-orm pg # Install development dependencies npm install -D drizzle-kit
Database Connection Setup
import { drizzle } from 'drizzle-orm/node-postgres'; import { Pool } from 'pg'; const pool = new Pool({ host: process.env.DB_HOST || 'localhost', port: parseInt(process.env.DB_PORT || '5432'), user: process.env.DB_USER || 'postgres', password: process.env.DB_PASSWORD, database: process.env.DB_NAME, }); const db = drizzle(pool);
Schema Definition and Management
Creating Database Schemas
import { pgTable, serial, text, timestamp, boolean } from 'drizzle-orm/pg-core'; export const users = pgTable('users', { id: serial('id').primaryKey(), name: text('name').notNull(), email: text('email').notNull().unique(), password: text('password').notNull(), createdAt: timestamp('created_at').defaultNow(), isActive: boolean('is_active').default(true), });
Essential CRUD Operations
Create Operations
const insertUser = async (userData: NewUser) => { const newUser = await db.insert(users) .values(userData) .returning(); return newUser[0]; };
Read Operations
// Fetch all users with pagination const getUsers = async (page: number, limit: number) => { return await db.select() .from(users) .limit(limit) .offset((page - 1) * limit); }; // Get user by ID const getUserById = async (userId: number) => { return await db.select() .from(users) .where(eq(users.id, userId)) .limit(1); };
Update Operations
const updateUser = async (userId: number, userData: Partial<User>) => { return await db.update(users) .set(userData) .where(eq(users.id, userId)) .returning(); };
Delete Operations
const deleteUser = async (userId: number) => { return await db.delete(users) .where(eq(users.id, userId)) .returning(); };
Advanced Features
Relationship Management
import { relations } from 'drizzle-orm'; // Define posts table export const posts = pgTable('posts', { id: serial('id').primaryKey(), title: text('title').notNull(), content: text('content').notNull(), userId: integer('user_id').references(() => users.id), }); // Define relationships export const usersRelations = relations(users, ({ many }) => ({ posts: many(posts), }));
Transaction Handling
const createUserWithPosts = async (userData: NewUser, posts: NewPost[]) => { return await db.transaction(async (tx) => { const user = await tx.insert(users).values(userData).returning(); const userPosts = posts.map(post => ({ ...post, userId: user[0].id })); await tx.insert(posts).values(userPosts); return user[0]; }); };
Performance Optimization Techniques
Query Optimization
// Selective column querying const getUserNames = await db .select({ id: users.id, name: users.name }) .from(users); // Index utilization export const users = pgTable('users', { email: text('email').notNull(), }, (table) => ({ emailIdx: index('email_idx').on(table.email), }));
Connection Pooling
const optimizedPool = new Pool({ max: 20, // maximum pool size idleTimeoutMillis: 30000, connectionTimeoutMillis: 2000, });
Database Migration Management
# Generate migration files npm run drizzle-kit generate:pg # Apply migrations npm run drizzle-kit push:pg
Best Practices and Design Patterns
Schema Validation
import { createInsertSchema } from 'drizzle-zod'; const insertUserSchema = createInsertSchema(users); const validateUser = (data: unknown) => insertUserSchema.parse(data);
Error Handling
try { await db.insert(users).values(userData); } catch (error) { if (error instanceof UniqueConstraintViolationError) { // Handle unique constraint violation } // Handle other errors }
Conclusion
Drizzle ORM represents a significant advancement in TypeScript ORM technology, offering developers a powerful yet intuitive tool for database operations. Its type-safe approach, combined with excellent performance characteristics, makes it an outstanding choice for modern web applications.
The framework's focus on developer experience, coupled with its robust feature set, positions it as a leading solution for projects requiring reliable and efficient database interactions. Whether you're building a small application or a large-scale system, Drizzle ORM provides the flexibility and functionality needed for success.
Keywords: TypeScript ORM, Drizzle ORM, database management, type-safe ORM, PostgreSQL, Node.js, database migrations, SQL query builder, TypeScript database, modern ORM